Today, we will provide an example of how we can get image pixels and how we can change them using the Pillow python library. You can find a short tutorial in Pillow here.
Usually, the images follow the RGB color model which means that every pixel is a vector of 3-D, where each position refers to the R (Red), G (Green) and B (Blue) respectively, each one taking values from 0-255. There is also the RGBA where we add another dimension called “alpha” which indicates how opaque each pixel is, taking values from 0-255 as well. Finally, there are the Grayscale images where each pixel is 1-D taking values from 0-255.
You should load the following libraries for the following examples:
import PIL from PIL import Image import requests from io import BytesIO from PIL import ImageFilter from PIL import ImageEnhance from IPython.display import display import numpy as np
Q: How to get the “mode” of the image (i.e. RGB, RGBA, L)?
URL = "https://www.timeanddate.com/scripts/cityog.php?title=Current%20Local%20Time%20in&city=New%20York&state=New%20York&country=USA&image=new-york1" response = requests.get(URL) img = Image.open(BytesIO(response.content)) # get the mode of the image img.mode # it returns 'rgb' # create a grayscale image grayimg = img.convert('L') grayimg.mode # it returns 'L' which means grayscale
Q: How to get the pixel of an image?
The command that we use is the “getpixel((x1,x2))”
# get the pixel of the top left corner of the RGB image img.getpixel((0,0)) # we get (44, 110, 160) since it is an RGB we expected a tuple of 3-D taking values from 0-255 # get the pixel of the top left corner of the grayscale image grayimg.getpixel((0,0)) # it returns 95 , as we expected we got only one element within the range of 0-255
Q: How to change a pixel of an image?
The command that we use it the “putpixel((x1,x2), value)”
# change the top left cornee pixel to white of the RBG Image img.putpixel( (0,0), (0,0,0) ) # change the top left corner pixel to white of the grayscale image grayimg.putpixel( (0,0), 0 )
Iterate over Image Pixels
Let’s give an example of Iterating over Image Pixels and based on a threshold to change the image color to white or black. For convenience our function will consider only grayscale images but you can easily extend it to RGA.
def binarize(image_to_transform, threshold): # now, lets convert that image to a single greyscale image using convert() output_image=image_to_transform.convert("L") # the threshold value is usually provided as a number between 0 and 255, which # is the number of bits in a byte. # the algorithm for the binarization is pretty simple, go through every pixel in the # image and, if it's greater than the threshold, turn it all the way up (255), and # if it's lower than the threshold, turn it all the way down (0). # so lets write this in code. First, we need to iterate over all of the pixels in the # image we want to work with for x in range(output_image.width): for y in range(output_image.height): # for the given pixel at w,h, lets check its value against the threshold if output_image.getpixel((x,y))< threshold: #note that the first parameter is actually a tuple object # lets set this to zero output_image.putpixel( (x,y), 0 ) else: # otherwise lets set this to 255 output_image.putpixel( (x,y), 255 ) #now we just return the new image return output_image binarize(img, 100)
For comparison we represent the Modified with a threshold of 100 and the Original!
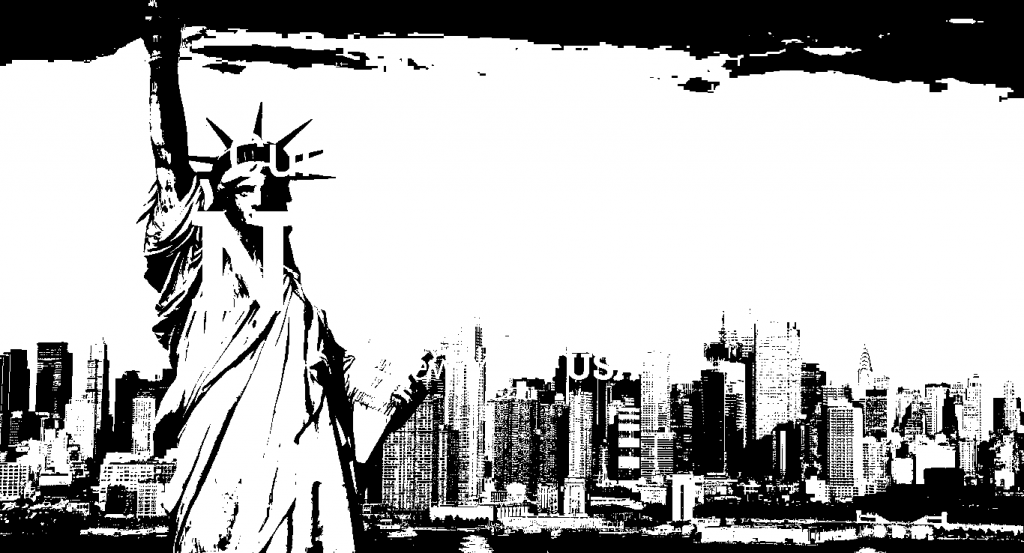

We can change also random pixels with random colors. The function below takes as an input the percentage of the pixels that are going to be changed with another random color from the RGB spectrum.
def random_image(image_to_transform, threshold): # convert any image to RGB output_image=image_to_transform.convert("RGB") # The threshold is the percentage of random pixels to generate for x in range(output_image.width): for y in range(output_image.height): if float(np.random.rand(1))<threshold: output_image.putpixel( (x,y), tuple(np.random.choice(range(255), 3, replace=True)) ) return output_image random_image(img, 0.2)
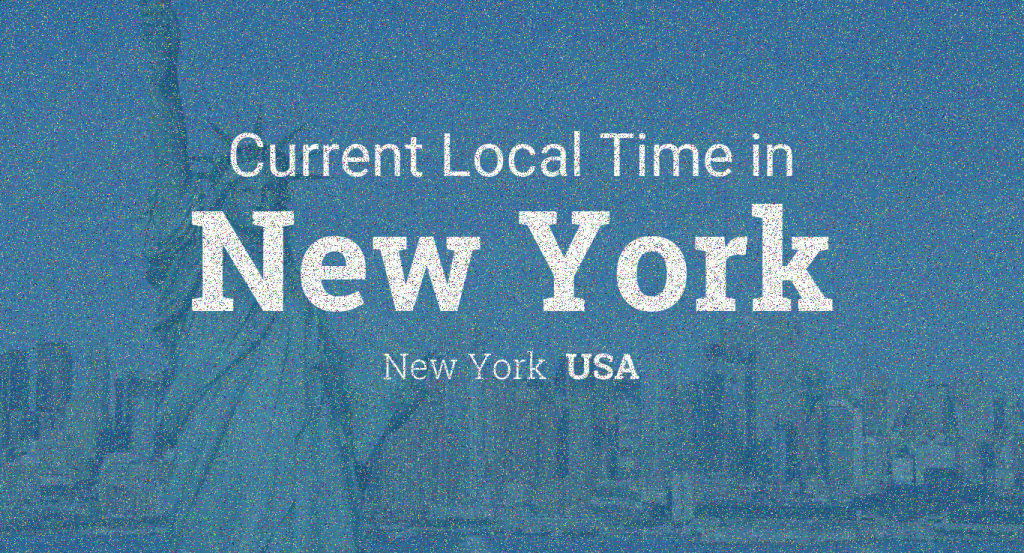
Related Articles
- Image Processing In OpenCV
- How to Extract Text From Images
- Get the Dominant Colors of an Image with K-means
- Most Dominant Color of an Image
- Blending Images with OpenCV
More Data Science Hacks?
You can follow us on Medium for more Data Science Hacks
4 thoughts on “Iterate over Image Pixels”
Thіs paragraph wilⅼ assist the internet users for creating new
blog or even a blog from start to end.
this is a really good content